Hey everyone. I'm currently using the ITaskbarList3 interface to reporting progress and visual present it in the taskbar for the application.
https://learn.microsoft.com/en-us/windows/win32/api/shobjidl_core/nn-shobjidl_core-itaskbarlist3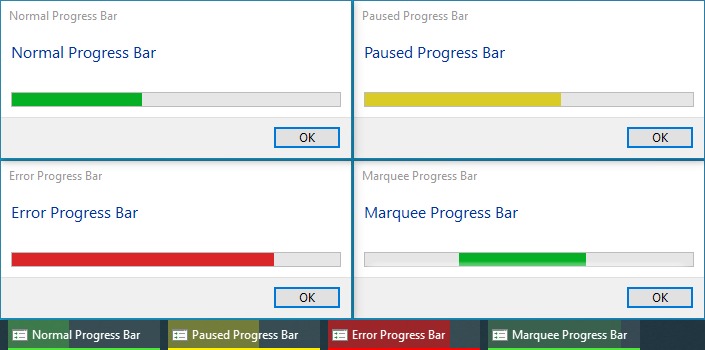
#include <shobjidl.h>
#pragma comment(lib, "user32.lib")
#pragma comment(lib, "comctl32.lib")
#pragma comment(lib, "ole32.lib")
WM_INITDIALOG:
CoInitialize(NULL);
ITaskbarList3 *pTaskbarList;
CoCreateInstance(&CLSID_TaskbarList, NULL, CLSCTX_INPROC_SERVER, &IID_ITaskbarList3, (void**)&pTaskbarList);
HWND hDlg = CreateDialog(GetModuleHandle(NULL), MAKEINTRESOURCE(DLG_MAIN), NULL, NULL);
// Set the taskbar progress value (example: 50%)
pTaskbarList->lpVtbl->SetProgressValue(pTaskbarList, hDlg, 50, 100);
// Perform other tasks...
// Clean up
pTaskbarList->lpVtbl->Release(pTaskbarList);
CoUninitialize();
Now it works fine, but it launches a new window to do it. How do I get progress on the main window dialog? It launches 2 windows, which is not desired.
Thank you!
EDIT:Sorry, I solved the issue. It was a matter of calling inside APIENTRY wWinMain(...)
...
HWND hMainWnd = CreateDialog(hInstance, MAKEINTRESOURCE(DLG_MAIN), NULL, (DLGPROC)MainDlgProc);
if (hMainWnd == NULL)
{
// Handle error if the dialog creation fails
MessageBox(NULL, L"Failed to create the main dialog", L"Error", MB_OK | MB_ICONERROR);
return 0;
}
// Initialize COM for the taskbar progress
CoInitialize(NULL);
ITaskbarList3 *pTaskbarList;
// Create an instance of the taskbar list
if (SUCCEEDED(CoCreateInstance(&CLSID_TaskbarList, NULL, CLSCTX_INPROC_SERVER, &IID_ITaskbarList3, (void**)&pTaskbarList)))
{
// Register the "TaskbarButtonCreated" message
UINT uTaskbarCreatedMsg = RegisterWindowMessage(L"TaskbarButtonCreated");
// Allow the main window to receive the "TaskbarButtonCreated" message
ChangeWindowMessageFilterEx(hMainWnd, uTaskbarCreatedMsg, MSGFLT_ALLOW, NULL);
// Set the taskbar progress value (example: 50%)
pTaskbarList->lpVtbl->SetProgressValue(pTaskbarList, hMainWnd, 50, 100);
// Perform other tasks...
// Clean up
pTaskbarList->lpVtbl->Release(pTaskbarList);
}
// Message loop
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// Uninitialize COM
CoUninitialize();
return msg.wParam;
}
------------------
UPDATE:Better solution. This uses ITaskbarList3 interface.
typedef enum {
PS_None,
PS_Normal,
PS_Paused,
PS_Error,
PS_Indeterminate
} ProgressStates;
void UpdateTaskbarProgress(HWND hWnd, ProgressStates state, int progressValue) {
ITaskbarList3 *taskbar;
if (CoCreateInstance(&CLSID_TaskbarList, NULL, CLSCTX_INPROC_SERVER, &IID_ITaskbarList3, (void**)&taskbar) != S_OK) {
return;
}
taskbar->lpVtbl->HrInit(taskbar);
TBPFLAG flags = TBPF_NOPROGRESS;
switch (state) {
case PS_Normal:
flags = TBPF_NORMAL;
break;
case PS_Paused:
flags = TBPF_PAUSED;
break;
case PS_Error:
flags = TBPF_ERROR;
break;
case PS_Indeterminate:
flags = TBPF_INDETERMINATE;
break;
}
taskbar->lpVtbl->SetProgressState(taskbar, hWnd, flags);
if (state == PS_Normal) {
taskbar->lpVtbl->SetProgressValue(taskbar, hWnd, progressValue, 100);
}
taskbar->lpVtbl->Release(taskbar);
}
Working as expected, with no issues

Credits to:
https://github.com/CyberSys/qutimCode is google cached (file does not exist anymore on the GIT link). Converted to C (above).
ITaskbarList4 interface appears to support adding buttons and things inside. I'll be testing this.
For example:
taskbar->lpVtbl->AddThumbBarButton(taskbar, hWnd, /* Button ID */ 1, THBF_ENABLED, NULL);